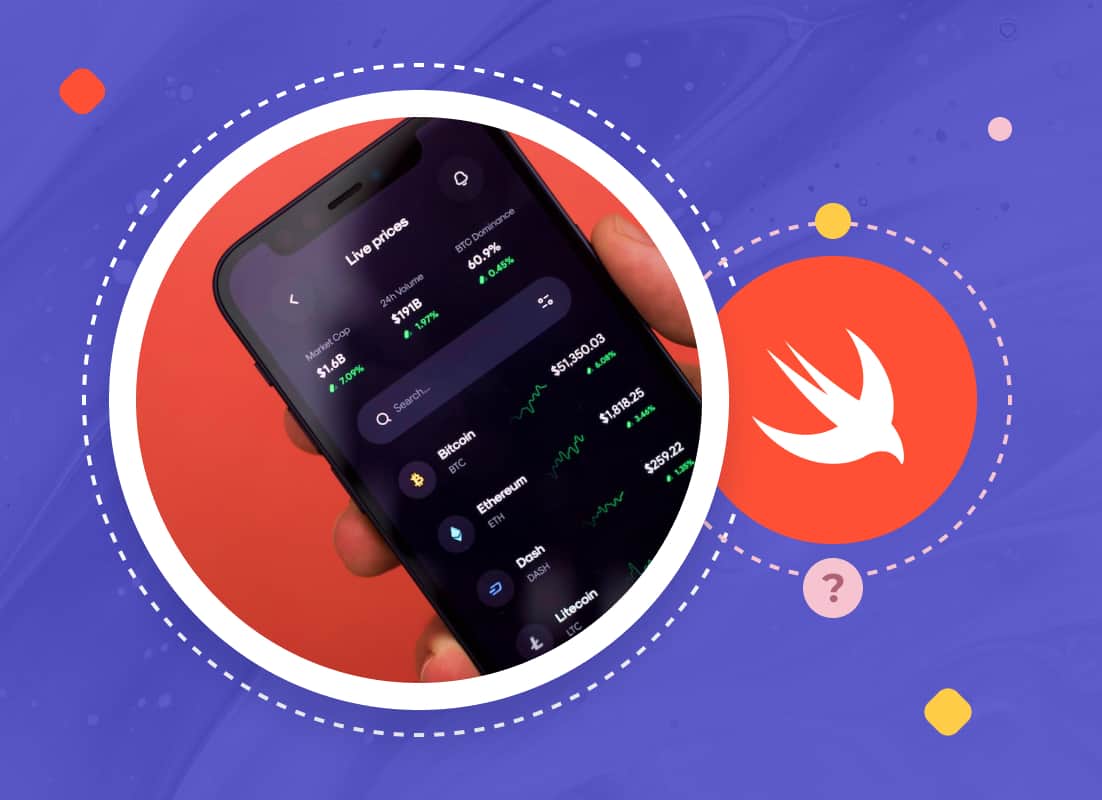
28 min read
How to Identify the iOS Swift Developer’s Level? Swift Interview Questions and Answers to Check a Candidate
Introduction
Swift is the most frequently used programming language released by Apple for building iOS native apps. Swift was created as a replacement for Apple’s previous Objective-C language and includes modern features that its predecessor lacked.
It is faster in execution, safer in terms of types prevention and memory management, as well as more powerful and less susceptible to bugs. This programming language is easy-to-use either for both newcomers or masters in programming.
There are a bunch of skills that determine beginner Swift developers from experts. In this article, you’ll find a list of criteria in a form of iOS Swift algorithm interview questions and answers for hiring companies to set a framework for interviewing.
The questions are broken down into categories: from the easiest ones aimed at junior-level engineers to iOS developer interview questions for experienced swift developers. Such a structure will help the employers determine the level of candidates and therefore, reduce risks when choosing an employee.
Swift Developers Required Skills in a Nutshell
The skillset presented below is about the ideal Swift language programmer. Use it as the directory.
- Exhaustive knowledge of Swift programming language and its ecosystem;
- Sufficient awareness of Xcode;
- Profound knowledge in Cocoa, Cocoa Touch;
- Great insight into object-oriented programming;
- Awareness in multi-paradigm programming languages (e.g. Python, JavaScript, etc.);
- In-depth knowledge of memory management and multi-threading overall (in languages such as Swift, Java, etc.);
- Good working knowledge of C-based libraries;
- Deep knowledge of test automation frameworks and most used DevOps tools;
- Vast experience in most commonly used RDBMSs (e.g. NoSQL, embedded, key-value pairs databases, etc.);
- Long-term experience in developing RESTful APIs;
- Experience in cloud computing platforms;
- Familiarity with tools such as GitHub;
- Great understanding of continuous integration (CI) and continuous delivery (CD) concepts;
- Familiarity with UI design incl. Apple’s “Human Interface Guidelines”.
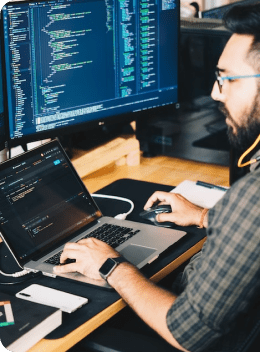
Swift Technical Interview Questions and Answers Collection
iOS Swift Basic Interview Questions and Answers for Junior-level Programmers
- What is Swift?
Answer: Swift is a programming language designed specifically for building iOS native apps for both smartphones and desktops (for iPadOS, macOS, tvOS, and watchOS). It was created by Apple together with the open-source community of experts (both inside Apple and external developers).
- List the pros and cons of using Swift.
Answer:
Pros:
- Fast execution speed thanks to the source code compiled then remains in a form of native machine code instructions.
- Type safety. The language is able to prevent typos.
- Memory safety. Swift prevents the variables from being initialized before they’re used to avoid program crashes. It takes less time to detect flaws with a shorter feedback loop.
- Interoperability with Objective-C. Projects written in Objective-C or C++ are still applicable to Swift.
- Ease of maintenance. Swift blends two Objective-C files — header (.m) and implementation (.h) — into one (.swift) whereas Objective-C itself operates on two separate files.
- Effective memory management.
- Memory usage is managed automatically by means of the ARC tool (Automatic Reference Counting) that detects and deletes unused class instances from the workspace.
- ABI (Application Binary Interface) stability. The stability of ABI provides binary compliance for apps and libraries across different Swift versions. Without stable ABI, an attempt to update the current app code to a newer Swift version might end up with a program crash. That happens because when ABI is not stable, each app built is related to its own Swift Dynamic Library. And since the ABI is not incorporated in iOS overall (not stable), but in each specific app (each specific Swift version has its own version of ABI), the OS would need to include a copy of every library compiled with every version of Swift. But when ABI is integrated into iOS, each newer version of Swift manages to access all the old compiled binaries. So the app built in one Swift version is able to communicate with a library built in another version. The ABI stability means that ABI is compliant with all the existing Swift versions and therefore, each app upgrade to a newer version of Swift happens consistently and smoothly. ABI stability was fine-tuned in the Swift 5.1 version.
- Optionals. Optional value is a type that represents either a wrapped value or the absence of a value (nil). With the Null Pointers, optionals protect values inside and therefore help to avoid application crashes. It’s required to unwrap optionals to ensure that the program doesn’t crash. Variable is declared with a ‘?’ mark.
- Better user experience. It takes less time to install and less device storage to keep apps based on Swift.
Cons:
- Relatively new language. Released in 2014, Swift can be considered a freshman, and its rapid growth might entail some inconveniences. The language has a limited set of tools and libraries. The community of experienced Swift developers is not that broad to help emerging Swift developers with the issues they tackle.
- Binary compatibility issue is not resolved completely. Code written in different versions of Swift can still malfunction despite stabilizing ABI in Swift 5.1. Objective C developers might have generated their code as a static library and built it into their projects as dependencies, which was impossible in Swift before the ABI was stabilized. Stable ABI made this possible, yet integrating dependencies is still an issue.
- Limited reflection.
Reflection is a regular programming language feature that enables the program to track down and amend its execution dynamically at runtime. Swift, in its turn, is not capable of altering the behavior of an enclosure of code providing view-only access to the object’s type or properties. This feature is called Mirror in Swift.
- What is Dictionary in Swift?
Answer: Dictionary in Swift is a basic data type in Swift that represents an unordered collection of key-value pairs. Accessing a value is possible with a unique key to each value linked to.
- Explain Enum in Swift?
Answer: Enum (or Enumeration) in Swift is a type of variable used to connect related values (e.g. Up and Down, Left and Right, True or False, Hot and Cold, etc.) for the purpose of type safety in coding. You can have more than 2 options to tie in the Enum (e.g. low, medium, high, extreme).
- Summarize the main differences between a structure and a class.
Answer:
Differences:
- Classes are reference types and structure data types operate as value types.
- Classes are able to inherit characteristics from other classes and structures don’t have inheritance functions at all.
- Class’s property can be mutated, but there is no mutability in structure sets.
- Classes support typecasting, but structures don’t.
- Class can be deinitialized with deinit() function before it’s destroyed which is impossible for structs.
- What are the basic data types in Swift?
Answer:
Data Type | What it does |
Int (Integer) | Stores integer value represented by whole numbers without fractions, e.g. 1, -3, 54, -115, etc. Can be a signed integer and unsigned integer. Size: can be 8, 16, 32, and 64-bit. Range (max and min values Int can store): ▫ Int: -128 to 127 (for 8-bit platform), -2^15 to 2^15-1 (for 16-bit platform), -2^31 to 2^31-1 (for 32-bit platform), and -2^63 to 2^63-1 (for 64-bit platform). ▫ UInt32: 0 to 2^32. ▫ UInt64: 0 to 2^64. Variable is declared with the Int keyword (more specifically “Int” for signed integer and “UInt” for unsigned integer). |
Double | Used for 64-bit floating-point numbers represented by large decimals with up to 15 decimal places, e.g. 2.700349234763, 0.86387234726, etc. Stores data with more precision. Range: 2.3E-308 to 1.7E+308. Variable is declared with the Double keyword. |
Float | Used for 64-bit floating-point numbers represented by shorter decimals with up to 6 decimal places, e.g. 3.98, 10.15, -45.34, etc. Stores data with less precision. Range: 1.2E-38 to 3.4E+38. Variable is declared with the Float keyword. |
Bool (Boolean) | Stores Boolean value. Represented by only 2 values: true and false. Variable is declared with the Bool keyword. |
String (String literals) | Represents textual data in quotation marks. Variable is declared with the String keyword, e.g. var confession: String = “I love you” (Output: I love you). |
Arrays (Array literal) | Represents an ordered collection of values of an equal type. Variable is declared with a list of values in square brackets, e.g. var fruit = [apple, peach, pear, banana]. |
Dictionaries | Represents an unordered collection of values of a specific type linked by a unique key. Variable is declared with the Dict keyword, e.g. var dict = [“key”: “value”]. |
- What are some common features in Swift structures and Swift classes?
Answer:
Common features:
- Both structures and classes define attributes for storing values and functions.
- They can create initializers to re-establish their initial state with init() function.
- Both may be extended with extensions.
- Structures and classes are able to collaborate with generics and generate adaptable and reusable types.
- Classes, as well as structures follow protocols.
- What’s the difference between == and ===?
Answer:
The equality operator (==) compares value types to check if two values are equal.
Identity operator (===) compares reference types to check if the two references link to the same object.
- Consider the following:
struct Tutorial { var difficulty: Int = 1 } var tutorial1 = Tutorial() var tutorial2 = tutorial1 tutorial2.difficulty = 2
What are the values of tutorial1.difficulty and tutorial2.difficulty? Would this be any different if the Tutorial was a class? Why or why not?
Answer:
tutorial1.difficulty is 1, whereas tutorial2.difficulty is 2.
Structures in Swift are value types. You copy value types by value rather than reference. The following code creates a copy of tutorial1 and assigns it to tutorial2:
var tutorial2 = tutorial1
A change to tutorial2 is not reflected in tutorial1.
If Tutorial were a class, both tutorial1.difficulty and tutorial2.difficulty would be 2. Classes in Swift are reference types. When you change a property of tutorial1, you’ll see it reflected in tutorial2 and vice versa.
- This complicated code sorts an array of names alphabetically.
var animals = ["fish", "cat", "chicken", "dog"] animals.sort { (one: String, two: String) -> Bool in return one < two } print(animals)
Simplify the closure as much as you can
Answer:
The type inference system automatically calculates both the type of the parameters in the closure and the return type so that you can get rid of them:
animals.sort { (one, two) in return one < two }
You can substitute the $i notation for the parameter names:
animals.sort { return $0 < $1 }
In single statement closures, you can omit the return keyword. The value of the last statement becomes the return value of the closure:
animals.sort { $0 < $1 }
Finally, since Swift knows that the elements of the array conform to Equatable, you can simply write:
animals.sort(by: <)
Swift Coding Interview Questions and Answers for Middle-level Programmers
- What’s the difference between Self vs self?
Answer:
“Self” and “self” are used in writing Protocols and Extensions.
Uppercase “Self” is a type (basically the current type in the current context). Capitalized Self can refer to any current type that complies with the protocol (e.g. Int or String).
Lowercase “self” is the object (“instance”). It can represent a struct, class, or property indicating whatever current object of a class (or struct, within that class. It indicates the value inside that type, e.g. textual data (“hello”), numbers (852), etc.
- When to use strong, weak, and unowned references?
Answer:
- In case you’re dealing with Structs or Enums, the ARC doesn’t perform memory management for them. So for such variables and constants, there’s no need to specify weak or unowned references.
- Strong references are utilized in hierarchical relationships with the identified owner and the objects it possesses, from the owner to its object and not the opposite way (e.g. a parent references the child).
- If the connection between two instances is not required, then one of them should have a weak reference to the other one.
- If two instances can’t exist without each other, then the instance with the compulsory dependency needs to get an unowned reference with regard to the other instance.
- What’s the difference between nil and .none?
Answer: They are equivalent and have no differences (nil == .none). Nil is more commonly used than .none.
- When to use a set rather than an array in Swift?
Answer:
Choose a set instead of an array if all the following conditions are met:
- An item is supposed to be utilized once only (sets do not accept duplicates).
- The order of the items in a set doesn’t matter.
- APIs use is not required (API requires using arrays only).
- You store Hashable types, your personal or one of Swift types such as integers or strings (sets use hash values for fast items search).
- Constant declaration in Objective-C is: const int number = 0;
Swift equivalent: let number = 0
What’s the difference?
Answer: “Const” is a variable initialized during compilation with a value or expression that must be settled at that same time of compilation.
The immutable declared with “let” is a constant defined at the time of execution. It can be initialized with a static or dynamic expression. It enables that kind of declaration:
let higherNumber = number + 5
It’s possible to assign its value only once.
- Explain the common execution states for a swift iOS App.
Answer:
Execution state | What it means |
Not running | the application is not launched, OR. the code is not being executed or stopped, and the app is completely off. |
Inactive | the app works in the background, yet can’t receive events. |
Active | the app works in the background and can receive events (primary state of execution). |
Background | the app works in the background and can execute the code in the background, as well. |
Suspended | the running app is in the background state, the system stops this app, so the app can’t execute the code. |
- Is it possible to add a stored property to a type by using an extension?
Answer: That’s not possible. The extension can help you add new behavior to an already existing type, but not by modifying the type per se and its interface. Adding stored property requires more memory space for storing new values, which cannot be done using the extension.
- State your understanding of core data.
Answer: Core Data is a framework for object graph management, object lifecycle maintenance, and managing model layer objects in iOS apps. It also serves as a tool for filtering, modifying, storing, grouping, and tracking data. With Core Data, you can link objects in the app to table records in the database (without learning SQL).
- Rewrite this code in a Swift way
let list = [Int](1...5) var arrayOfTuples = [(Int, Int)]() for (index, element) in list.enumerated() { arrayOfTuples += [(index, element)] } print(arrayOfTuples) // prints [(0, 1), (1, 2), (2, 3), (3, 4), (4, 5)]
Answer:
Yeap, the way to do it is:
let list = [Int](1...5) let arrayOfTuples = Array(list.enumerated()) print(arrayOfTuples) // prints [(offset: 0, element: 1), (offset: 1, element: 2), (offset: 2, element: 3), (offset: 3, element: 4), (offset: 4, element: 5)]
or with map:
let list = [Int](1...5) let arrayOfDictionaries = list.enumerated().map { (a, b) in return [a : b] } print(arrayOfDictionaries) // prints [[0: 1], [1: 2], [2: 3], [3: 4], [4: 5]]
- What is a good use case for an inout parameter?
Answer:
Function parameters are constants by default. Trying to change the value of a function parameter from within the body of that function results in a compile-time error.
inout means that modifying the local variable will also modify the passed-in parameters. Without it, the passed-in parameters will remain at the same value. Trying to think of reference type when you are using inout and value type without using it.
- A good use case will be a swap function that will modify the passed-in parameters.
- Consider removing the overhead of copying as well. If you have a function that takes a somewhat memory-wise large value type as an argument (say, a large structure type) and that returns the same type, and finally where the function return is always used just to replace the caller argument, then inout is to prefer as an associated function parameter.
struct MyStruct { private var myInt: Int = 1 // ... lots and lots of stored properties mutating func increaseMyInt() { myInt += 1 } } /* call to function _copies_ argument to function property 'myHugeStruct' (copy 1) function property is mutated function returns a copy of mutated property to caller (copy 2) */ func myFunc(var myHugeStruct: MyStruct) -> MyStruct { myHugeStruct.increaseMyInt() return myHugeStruct } /* call-by-reference, no value copy overhead due to inout opimization */ func myFuncWithLessCopyOverhead(inout myHugeStruct: MyStruct) { myHugeStruct.increaseMyInt() } var a = MyStruct() a = myFunc(a) // copy, copy: overhead myFuncWithLessCopyOverhead(&a) // call by reference: no memory reallocation
Advanced Swift Programming Interview Questions for Senior Developers
The hardest task for the hiring company is to test senior-level developers. Forming the most advanced Swift interview questions and answering them is a challenge for expert-level programmers only.
- What are the advantages of using Combine instead of traditional event-handling architectures like delegates and callbacks?
Answer:
There are several advantages to using Combine instead of traditional event-handling architectures like delegates and callbacks.
First, Combine allows you to declaratively express your event-handling logic, which can make your code more readable and easier to understand.
Second, Combine can help you avoid common pitfalls associated with traditional event-handling architectures, such as race conditions and memory leaks.
Finally, Combine provides a number of built-in operators and publishers that can make it easier to work with asynchronous data sources.
- What is the use of deinit in swift?
Answer:
Deinitialization in Swift is the method of deallocating or cleaning up unused class instance objects in order to free up memory space used by machine resources for better memory management. Until a class instance is deallocated, the deinitialization process is usually named.
- Can you describe a circular reference in Swift? How can you solve it?
Answer:
A circular reference happens when two instances hold a strong reference to each other, causing a memory leak because neither of the two instances will ever be deallocated. The reason is that you cannot deallocate an instance as long as there’s a strong reference to it, but each instance keeps the other alive because of its strong reference.
You’d solve the problem by breaking the strong circular reference by replacing one of the strong references with a weak or unowned reference.
- How to append one array to another array in Swift?
var first = ["John", "Paul"] let second = ["George", "Ringo"]
Answer:
- One option for joining arrays is the append(contentsOf:) method, used like this:
first.append(contentsOf: second)
- Another option is using the += operator, which is overloaded for arrays to combine elements:
first += second
- The third option is to use a regular + to create a new array by combining two others:
let third = first + second
All three options produce the same resulting array.
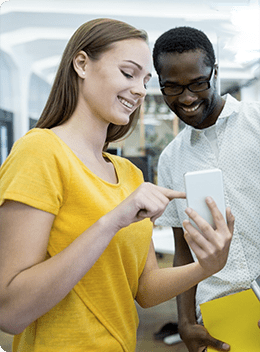
Soft Skills
Soft skills are the skills that are applicable to any profession and form the emotional side of a personality. In the IT business domain, soft skills are usually opposed to hard skills as those which have nothing in common with engineering competencies.
We’ve divided all the necessary soft skills into two categories. The first one contains those skills that are valuable even for newbies but their role is increasing with the developer’s seniority level.
The second group of skills has little effect on junior- and middle-level developers. These personal qualities, however, become highly essential when we speak about senior-level developers, team leaders, and other employees who manage the staff.
Overall (the role of every single skill increases with the seniority level):
- Problem-solving skills (this isn’t about solving bugs found in the code)
- Critical thinking (an ability to make the right decisions and act under serious pressure and in critical obstacles)
- Communication (or social skills)
- Self-awareness (an ability to understand your own personal behavior aspects — values, beliefs, emotions — and control them)
- Self-learning (how you can research and understand unfamiliar concepts)
- Self-organization (how efficiently you can work without any supervision)
- Accountability (responsibility for the results of your actions)
- Coachability (an ability to perceive feedback and react to it adequately)
- Time management (a skill of advanced planning allowing you to make more in less time)
- Teamwork (an ability to efficiently collaborate with other people).
Some of the more specific soft skills for senior-level developers:
- Leadership (an ability to manage other people efficiently)
- Failure anticipation (a skill of understanding the impending problems before they even arrive)
- Escalation management (an ability to effectively communicate potential and current problems to upper management)
- Interviewer skills (a skill of arranging interviews and understanding the unvarnished picture of an interviewing person)
- Growth mindset (a tendency to take challenges as opportunities to become better and smarter by solving them).
How to test soft skills
The question isn’t so easy to be answered with a swoop. Asking straightforward questions like “Are you able to anticipate failures” will give you no desired understanding. The answers will highly likely be very expected: it’s easy to lie here but rather difficult to verify.
So, how to check the soft skills of a candidate in a more elegant way? First of all, choose several of the most important soft skills you’ll have to verify. It would be rather hard to check a full list of soft skills, and there is no need.
For each skill you’ve chosen, you must create a tricky question. Such questions must be as much unexpected for the interviewee as possible. As a result, they’ll be discouraged at the moment of their answer.
Don’t pay much attention to the words they’ll say. The thing you should look at is their immediate emotional reaction in stressful situations.
For example, ask something technical and then suddenly offer an interviewee to teach them a bit about the topic you’ve just raised. People with insufficient self-awareness, coachability, and other related soft skills will definitely show their frustration and unwillingness to be taught this unusual way.
Another example: offer an interviewee to play a role game. Within the game, they will explain any complex concept to a less experienced colleague.
The first reaction will show you the candidate’s attitude to unexpected communication sessions (people with communication difficulties will clearly show their frustration in the first moment after your offer). Once the interviewee agrees, start playing the most stupid trainee you can. Several dull questions in a row can show you how the candidate is handling teamwork, communication, coaching, and, in some aspects, emotional intelligence.
Wrapping Up
Let’s recap our findings:
- The higher level your new Swift developer must be, the more qualified another developer you should have to test their skills.
- The skills Swift developers must have varied by both their focus angle and depth.
- The more skillful developer is, the easier it is for them to avoid sharp questions within their answers.
- Examining soft skills is about producing unexpected situations and then checking the reaction.
- You can find more Swift interview questions by visiting GitHub.
Remote tech teams & the future of work blog
Remote tech teams & the future of work blog
Your form has been successfully submitted.